Zhenjing (Jerry) Yu
Scotty3D
C++, Computer Graphics
Scotty3D is the coursework project for 15-462/662(Computer Graphics) at Carnegie Mellon University. It is a 3D graphics software package including components for interactive mesh editing, realistic path tracing, and dynamic animation.
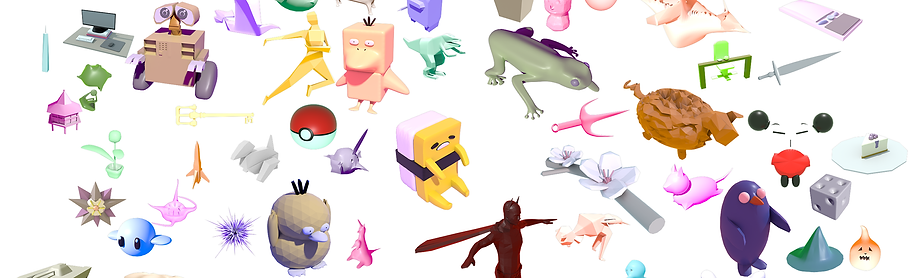
MeshEdit
MeshEdit is the first major component of Scotty3D, which performs 3D modeling, subdivision, and mesh processing. It enables the user to transform a simple cube model into beautiful, organic 3D surfaces described by high-quality polygon meshes. This tool can import, modify, and export industry-standard COLLADA files, allowing Scotty3D to interact with the broader ecosystem of computer graphics software.
I implemented most of the local mesh operations that Scotty3D supports, such as EdgeFlip, EdgeCollapse, VertextExtrude, FaceBevel, etc. It gives me a comprehensive understanding of the Halfedge data structure, which acts like “glue” connecting the different elements such as vertices, edges, and faces.
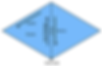
Apart from that, I also implemented some global remeshing algorithms including Catmull-Clark Subdivision, Isotropic Remeshing, and Simplification. These algorithms allow me to modify and optimize my models the same way as some of the well-known 3D software do.
Catmull-Clark Subdivision:
Isotropic Remeshing:
Simplification:

Pathtracer
PathTracer is (as the name suggests) a simple path tracer that can render scenes with global illumination. I implemented the BVH(Bounding Volume Hierarchy) algorithm as the data structure to store the mesh information and accelerate the frequent ray intersection query that path tracing requires.
The images below show the visualization of the bounding box hierarchy of a bunny model. The image from left to right indicates the bounding box at level 4, 7, and 10.

Also, the efficient BVH structure accelerates the visibility query for the bunny model from 2 minutes to less than 0.5 seconds on an RTX 3070 machine.
Later on, I implemented the global illumination algorithm using path tracing. I also applied BSDF for Lambertian, mirror, and glass material to test the correctness and efficiency of my algorithm.


Cornell box, with Lambertian BSDF
720p, 1024 samples per pixel
Cornell box, with mirror and glass BSDF
720p, 1024 samples per pixel
To optimize the path tracing algorithm even more, I implemented a basic importance sampling strategy - light sampling. The basic idea behind importance sampling is that for each point the algorithm prefers to shoot rays in the direction that has light sources because these are the samples that actually contribute to the final rendering result and therefore have better rendering quality even with fewer samples.

Without (left) and with (right) area light sampling (32 samples, max depth = 8):
Lastly, I implemented Environment Lighting, which is a new type of light source: an infinite environment light. An environment light is a light that supplies incident radiance from all directions on the sphere. The intensity of incoming light from each direction is defined by a texture map parameterized by phi and theta:
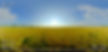
Pixels with higher luminance in the texture map will have a higher probability to be sampled from. For this purpose, I implemented an inversion sampling algorithm by calculating the luminance PDF and CDF of the texture map and then sample pixels based on the CDF.
​
The rendering results of Environment Lighting are shown below. The model in the right image is made by myself using the local and global mesh operations I implemented in MeshEdit.

